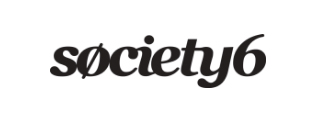
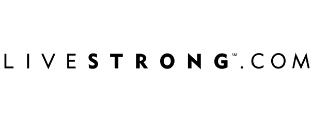
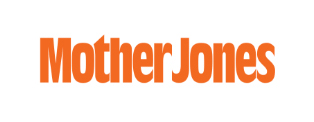
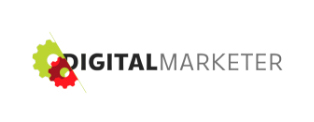
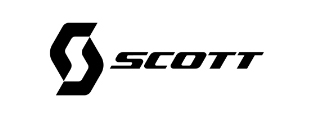
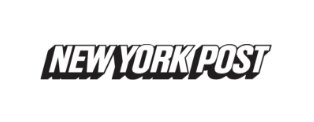
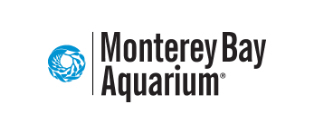
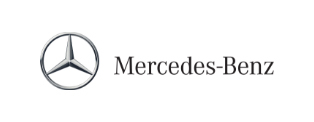
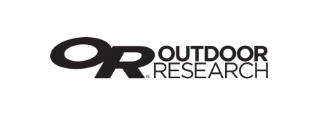
Flexible API Integrations Fit
for Every Application
JetSend’s on Stand-By, Ready to Implement with Any Application at Any Time.
1
var request = require(‘request’);
2
var options = {
3
‘method’: ‘POST’,
4
‘url’: ‘http://sandbox.jetsend.com:8080/v1/injection’,
5
headers’: {
6
‘Authorization’: ‘Basic Your Api Key’,
7
‘Content-Type’: [‘application/json’]
8
},
9
body: “{\
10
“from_email\”: \”From Email Address\”,
11
\”rcpt_to\”: [\”To Email Address\”],
12
\”subject\”:\”jetsend\”,
13
\”body\”: \”Hello jetsend!!!\”
14
}”
15
};
16
request(options, function (error, response) {
17
if (error) throw new Error(error);
18
console.log(response.body);
19
});
1
var request = require("request");
2
var options = {
3
method: 'POST',
4
url: 'https://staging.jetsend.com/api/v1/email',
5
headers: {
6
'content-type': 'application/json',
7
accept: 'application/json',
8
authorization: '[YOUR_API_KEY]'
9
},
10
body: {
11
from_address: '[FROM_EMAIL_ADDRESS]',
12
to_address: '[RECIPIENT_EMAIL_ADDRESS]',
13
subject: 'Welcome to Jetsend!',
14
tags: ['new-user', 'alert'],
15
tracking_domain: '[YOUR_VERIFIED_TRACKING_DOMAIN]',
16
body: '<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href=\'http://support.jetsend.com\'>JetSend Support</a></p>this is just a test email to test clicks and opens'
17
},
18
json: true
19
};
20
21
request(options, function (error, response, body) {
22
if (error) throw new Error(error);
23
console.log(body);
24
});
1
require 'uri'
2
require 'net/http'
3
require 'openssl'
4
5
url = URI("https://staging.jetsend.com/api/v1/email")
6
7
http = Net::HTTP.new(url.host, url.port)
8
http.use_ssl = true
9
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
10
11
request = Net::HTTP::Post.new(url)
12
request["content-type"] = 'application/json'
13
request["accept"] = 'application/json'
14
request["authorization"] = '[YOUR_API_KEY]'
15
request.body = "{\n\t\"from_address\": \"[FROM_EMAIL_ADDRESS]\",\n\t\"to_address\":
16
\"[RECIPIENT_EMAIL_ADDRESS]\",\n\t\"subject\": \"Welcome to Jetsend!\",\n\t\"tags\":
17
[\"new-user\",\"alert\"],\n\t\"tracking_domain\":
18
\"[YOUR_VERIFIED_TRACKING_DOMAIN]\",\n\t\"body\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email to test clicks and opens\"\n}\n"
19
20
response = http.request(request)
21
puts response.read_body
1
import http.client
2
3
conn = http.client.HTTPSConnection("staging.jetsend.com")
4
5
payload = "{\n\t\"from_address\": \"[FROM_EMAIL_ADDRESS]\",\n\t\"to_address\": \"[RECIPIENT_EMAIL_ADDRESS]\",\n\t\"subject\": \"Welcome to Jetsend!\",\n\t\"tags\": [\"new-user\",\"alert\"],\n\t\"tracking_domain\": \"[YOUR_VERIFIED_TRACKING_DOMAIN]\",\n\t\"body\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email to test clicks and opens\"\n}\n"
6
7
headers = {
8
'content-type': "application/json",
9
'accept': "application/json",
10
'authorization': "[YOUR_API_KEY]"
11
}
12
13
conn.request("POST", "/api/v1/email", payload, headers)
14
15
res = conn.getresponse()
16
data = res.read()
17
18
print(data.decode("utf-8"))
1
package main
2
3
import (
4
"fmt"
5
"strings"
6
"net/http"
7
"io/ioutil"
8
)
9
10
func main() {
11
url := "https://staging.jetsend.com/api/v1/email"
12
13
payload := strings.NewReader("{\n\t\"from_address\": \"[FROM_EMAIL_ADDRESS]\",\n\t\"to_address\": \"[RECIPIENT_EMAIL_ADDRESS]\",\n\t\"subject\": \"Welcome to Jetsend!\",\n\t\"tags\": [\"new-user\",\"alert\"],\n\t\"tracking_domain\": \"[YOUR_VERIFIED_TRACKING_DOMAIN]\",\n\t\"body\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email to test clicks and opens\"\n}\n")
14
15
req, _ := http.NewRequest("POST", url, payload)
16
17
req.Header.Add("content-type", "application/json")
18
req.Header.Add("accept", "application/json")
19
req.Header.Add("authorization", "[YOUR_API_KEY]")
20
21
res, _ := http.DefaultClient.Do(req)
22
23
defer res.Body.Close()
24
body, _ := ioutil.ReadAll(res.Body)
25
26
fmt.Println(res)
27
fmt.Println(string(body))
28
}
1
<?php
2
3
$request = new HttpRequest();
4
$request->setUrl('https://staging.jetsend.com/api/v1/email');
5
$request->setMethod(HTTP_METH_POST);
6
7
$request->setHeaders(array(
8
'content-type' => 'application/json',
9
'accept' => 'application/json',
10
'authorization' => '[YOUR_API_KEY]'
11
));
12
13
$request->setBody('{
14
"from_address": "[FROM_EMAIL_ADDRESS]",
15
"to_address": "[RECIPIENT_EMAIL_ADDRESS]",
16
"subject": "Welcome to Jetsend!",
17
"tags": ["new-user","alert"],
18
"tracking_domain": "[YOUR_VERIFIED_TRACKING_DOMAIN]",
19
"body": "<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href=\"http://support.jetsend.com\">JetSend Support</a></p>this is just a test email to test clicks and opens"
20
}
21
');
22
23
try {
24
$response = $request->send();
25
echo $response->getBody();
26
} catch (HttpException $ex) {
27
echo $ex;
28
}
1
var data = JSON.stringify({
2
"from_address": "[FROM_EMAIL_ADDRESS]",
3
"to_address": "[RECIPIENT_EMAIL_ADDRESS]",
4
"subject": "Welcome to Jetsend!",
5
"tags": [
6
"new-user",
7
"alert"
8
],
9
"tracking_domain": "[YOUR_VERIFIED_TRACKING_DOMAIN]",
10
"body": "<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email to test clicks and opens"
11
});
12
13
var xhr = new XMLHttpRequest();
14
xhr.withCredentials = true;
15
16
xhr.addEventListener("readystatechange", function () {
17
if (this.readyState === this.DONE) {
18
console.log(this.responseText);
19
}
20
});
21
22
xhr.open("POST", "https://staging.jetsend.com/api/v1/email");
23
xhr.setRequestHeader("content-type", "application/json");
24
xhr.setRequestHeader("accept", "application/json");
25
xhr.setRequestHeader("authorization", "[YOUR_API_KEY]");
26
27
xhr.send(data);
1
var client = new RestClient("https://staging.jetsend.com/api/v1/email");
2
var request = new RestRequest(Method.POST);
3
request.AddHeader("content-type", "application/json");
4
request.AddHeader("accept", "application/json");
5
request.AddHeader("authorization", "[YOUR_API_KEY]");
6
request.AddParameter("application/json", "{\n\t\"from_address\": \"[FROM_EMAIL_ADDRESS]\",\n\t\"to_address\": \"[RECIPIENT_EMAIL_ADDRESS]\",\n\t\"subject\": \"Welcome to Jetsend!\",\n\t\"tags\": [\"new-user\",\"alert\"],\n\t\"tracking_domain\": \"[YOUR_VERIFIED_TRACKING_DOMAIN]\",\n\t\"body\": \"<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email to test clicks and opens\"\n}\n", ParameterType.RequestBody);
7
IRestResponse response = client.Execute(request);
1
import Foundation
2
3
let headers = [
4
"content-type": "application/json",
5
"accept": "application/json",
6
"authorization": "[YOUR_API_KEY]"
7
]
8
let parameters = [
9
"from_address": "[FROM_EMAIL_ADDRESS]",
10
"to_address": "[RECIPIENT_EMAIL_ADDRESS]",
11
"subject": "Welcome to Jetsend!",
12
"tags": ["new-user", "alert"],
13
"tracking_domain": "[YOUR_VERIFIED_TRACKING_DOMAIN]",
14
"body": "<h2>Hello!</h2><p>You have successfully sent an email via JetSend API.</p><p>Click this link to check our help center page: <a href='http://support.jetsend.com'>JetSend Support</a></p>this is just a test email to test clicks and opens"
15
] as [String : Any]
16
17
let postData = JSONSerialization.data(withJSONObject: parameters, options: [])
18
19
let request = NSMutableURLRequest(url: NSURL(string:
20
"https://staging.jetsend.com/api/v1/email")! as URL,
21
cachePolicy: .useProtocolCachePolicy,
22
timeoutInterval: 10.0)
23
request.httpMethod = "POST"
24
request.allHTTPHeaderFields = headers
25
request.httpBody = postData as Data
26
27
let session = URLSession.shared
28
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
29
if (error != nil) {
30
print(error)
31
} else {
32
let httpResponse = response as? HTTPURLResponse
33
print(httpResponse)
34
}
35
})
36
37
dataTask.resume()
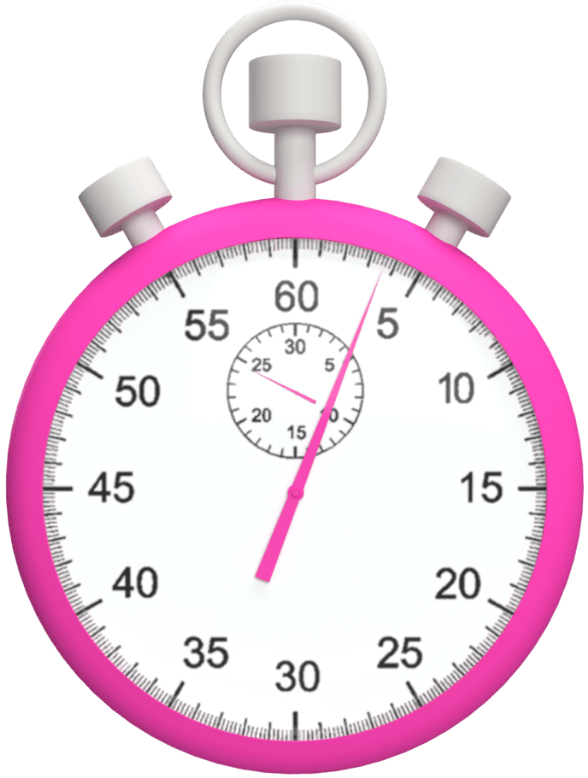
Timing is Everything!
JetSend follows the ‘5 seconds or less’ rule! Send speed is important, 70% of customers and users expect transactional emails to be delivered within 5 seconds of being triggered.